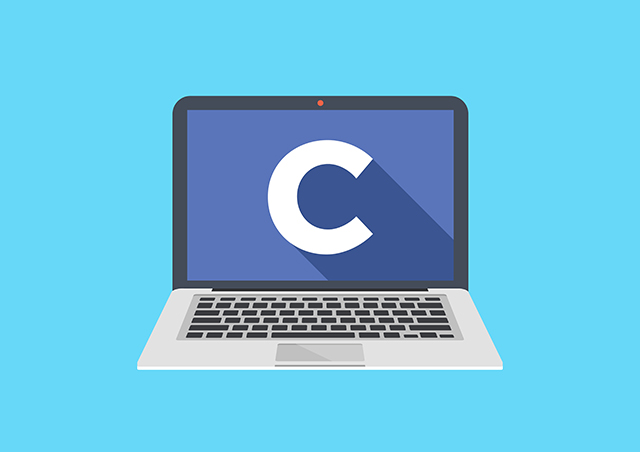
Hello Friend, Many of you were contacting me via social medias asking for C Program to Create a Student Database Using Structure. So here is the code to make a student database.
jp@jp-VirtualBox:~/$ ./a.out studentDB.txt
Enter the number of students:3
Enter student 1 details:
Name: Ram
Roll Number: 101
Age: 10
Mark1, Mark2, Mark3:100 100 100
Enter student 2 details:
Name: Sam
Roll Number: 102
Age: 10
Mark1, Mark2, Mark3:95 96 97
Enter student 3 details:
Name: Tom
Roll Number: 103
Age: 10
Mark1, Mark2, Mark3:95 97 99
Enter the desired student roll number:101
Details of Ram:
RollNo: 101
Age: 10
Mark1: 100
Mark2: 100
Mark3: 100
Average: 100.000000
Do you want to continue(1/0):1
Enter the desired student roll number:103
Details of Tom:
RollNo: 103
Age: 10
Mark1: 95
Mark2: 97
Mark3: 99
Average: 97.000000
Do you want to continue(1/0):0
jp@jp-VirtualBox:~/$ cat studentDB.txt
101 Ram 10 100 100 100 100.000000
102 Sam 10 95 96 97 96.000000 103 Tom 10 95 97 99 97.000000
Note:
./a.out studentDB.txt => passing file name(studentDB.txt) in command line.
./a.out studentDB.txt => passing file name(studentDB.txt) in command line.
Code:
- #include <stdio.h>
- #include <stdlib.h>
- #include <string.h>
- /* student structure */
- struct student {
- char name[32];
- int age, rollNo;
- int m1, m2, m3;
- float average;
- };
- int main(int argc, char *argv[]) {
- struct student s1;
- int i, n, m1, m2, m3, age, flag, rollNo;
- char name[32];
- float average;
- FILE *fp;
- /* open the given input file in write mode */
- fp = fopen(argv[1], "w");
- /* get the number of students from the user */
- printf("Enter the number of students:");
- scanf("%d", &n);
- /* get student information from user and write it to a file */
- for (i = 0; i < n; i++) {
- printf("\nEnter student %d details:\n", i + 1);
- getchar();
- printf("Name: ");
- fgets(name, 32, stdin);
- name[strlen(name) - 1] = '\0';
- printf("Roll Number: ");
- scanf("%d", &rollNo);
- printf("Age: ");
- scanf("%d", &age);
- printf("Mark1, Mark2, Mark3:");
- scanf("%d%d%d", &m1, &m2, &m3);
- average = (1.0 * (m1 + m2 + m3)) / 3;
- /* writing student information to a file */
- fprintf(fp, "%d %s %d %d %d %d %f\n",
- rollNo, name, age, m1, m2, m3, average);
- }
- /* closing the opened file */
- fclose(fp);
- /* opening the input file in read mode */
- fp = fopen(argv[1], "r");
- /*
- * fetches the desired student record from
- * file and displays it to the user
- */
- while (1) {
- printf("\nEnter the desired student roll number:");
- scanf("%d", &rollNo);
- flag = 0;
- while (!feof(fp)) {
- fscanf(fp, "%d %s %d %d %d %d %f", &s1.rollNo,
- s1.name, &s1.age, &s1.m1, &s1.m2,
- &s1.m3, &s1.average);
- if (s1.rollNo == rollNo) {
- printf("Details of %s:\n", s1.name);
- printf("RollNo: %d\n", s1.rollNo);
- printf("Age: %d\n", s1.age);
- printf("Mark1: %d\n", s1.m1);
- printf("Mark2: %d\n", s1.m2);
- printf("Mark3: %d\n", s1.m3);
- printf("Average: %f\n", s1.average);
- flag = 1;
- break;
- }
- }
- if (!flag) {
- printf("Record Not Found!!\n");
- }
- /* rewinding the file pointer to the start of file */
- rewind(fp);
- printf("Do you want to continue(1/0):");
- scanf("%d", &flag);
- if (!flag)
- break;
- }
- /* closing the file */
- fclose(fp);
- return(0);
- }
Output:
jp@jp-VirtualBox:~/$ ./a.out studentDB.txt
Enter the number of students:3
Enter student 1 details:
Name: Ram
Roll Number: 101
Age: 10
Mark1, Mark2, Mark3:100 100 100
Enter student 2 details:
Name: Sam
Roll Number: 102
Age: 10
Mark1, Mark2, Mark3:95 96 97
Enter student 3 details:
Name: Tom
Roll Number: 103
Age: 10
Mark1, Mark2, Mark3:95 97 99
Enter the desired student roll number:101
Details of Ram:
RollNo: 101
Age: 10
Mark1: 100
Mark2: 100
Mark3: 100
Average: 100.000000
Do you want to continue(1/0):1
Enter the desired student roll number:103
Details of Tom:
RollNo: 103
Age: 10
Mark1: 95
Mark2: 97
Mark3: 99
Average: 97.000000
Do you want to continue(1/0):0
jp@jp-VirtualBox:~/$ cat studentDB.txt
101 Ram 10 100 100 100 100.000000
102 Sam 10 95 96 97 96.000000 103 Tom 10 95 97 99 97.000000
0 Comments